Java do-while Loop
By Stephen Bucaro
A loop is a block of statements that are repeated. A loop may be repeated a fixed number of
times, repeat until some condition is met, or can loop indefinitely while the program is running.
There are three main loop control structures available in Java: for, do and while.
The do-while loop is similar to the while loop, except the conditional test is
performed after the execution of the code in the loops code block. Shown below is an example of
a do-while loop.
int iVal = 0;
do {
iVal++;
} while(iVal < 10);
The do-while loop starts out by executing the code inside the code block of the loop.
Inside the code block of the while loop, the value of iVal is incremented (increased) by 1.
After the code inside the code block is executed, the conditional test checks if the value in the
variable iVal is less than 10. While this condition is true, the do-while loop continues.
The value of iVal will be incremented each time the while condition is true.
On the tenth iteration through the loop, the value of iVal will not be less than 10, so the
condition in the while text will turn false and the loop will exit.
iVal | code block | result |
0 | inc iVal to 1 | true |
1 | inc iVal to 2 | true |
2 | inc iVal to 3 | true |
3 | inc iVal to 4 | true |
4 | inc iVal to 5 | true |
5 | inc iVal to 6 | true |
6 | inc iVal to 7 | true |
7 | inc iVal to 8 | true |
8 | inc iVal to 9 | true |
9 | inc iVal to 10 | false > exit loop |
Note: some important things about the do-while loop is that the statements within the
do-while code block are always executed at least once, and something must happen within the
code block to change the test variable or it will loop indefinitely.
So, the resulting function of this while loop example is to generate the numbers
1 through 10. Shown below is the complete code for an application that displays the output
in a program window.
import javax.swing.*;
public class doWhileLoop
{
public static void main(String s[])
{
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.setSize(300,300);
frame.setLocationRelativeTo(null);
frame.setTitle("Java do While Loop Test");
String outData = "";
int iVal = 0;
do {
iVal++;
String strI = String.valueOf(iVal);
outData += strI + " ";
} while(iVal < 10);
JTextArea myText = new JTextArea();
myText.setLineWrap(true);
myText.append(outData);
frame.add(myText);
frame.setVisible(true);
}
}
In the code above, the code in the do-while loop code block uses the valueOf method
to convert iVal to a string and then concatenates it onto the end of the string variable
outData. A JTextArea object named myTextis created, and the line
myText.append(outData) places the outData string in the JTextArea for
display in the program window.
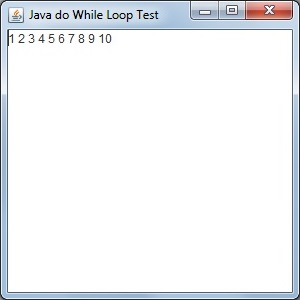
|