Locate Text Within Text in a Java Program
By Stephen Bucaro
In computer programming, a letter or digit is called a character and a collection of letters
or digits that make a phrase, sentence, or paragraph, is called a string. Java uses the String
object's .indexOf method to locate one string within another. The code shown below locates the
text "Teach Yourself Java" in the string "A good book to learn Java programming is Sam’s Teach Yourself
Java in 24 Hours".
import javax.swing.*;
public class textinText
{
public static void main(String s[])
{
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.setSize(300,300);
frame.setLocationRelativeTo(null);
frame.setTitle("Text in Text");
String fullText = "A good book to learn Java programming is Sams Teach Yourself Java in 24 Hours";
int loc = fullText.indexOf("Teach Yourself Java");
String result = "Substring starts at character " + loc;
JTextArea myText = new JTextArea();
myText.setLineWrap(true);
myText.append(result);
frame.add(myText);
frame.setVisible(true);
}
}
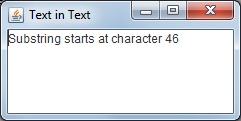
The .indexOf method returns the zero-based location where the substring begins, in other words the
first character in the source string is location 0. If the substring is not found in the source string,
.indexOf returns -1.
I generally hate it when people add a bunch of extra code to an example. It confuses things.
But in this case I've included the javax.swing library and added code to create a
JFrame and a JTextArea. If I didn't add this extra code, you would need to use
the System.out.print command to output text, and I don't think that's an interesting
or realistic way to learn Java programming.
In the code above, the line that starts with String fullText = declares a String object
named fullText and initializes it with the source string text. The next line uses the
.indexOf method to return the index of the substrting "Teach Yourself Java" within the
source string. The next line declares String object named result and initializes it
with the string "Substring starts at character " using the concatenate operator (+)
to paste that index on the end of the string.
A JTextArea object named myTextis created, and the line myText.append(result)
places the result string in the JTextArea for display in the program window.
If you're a beginner, for this example, I recommend downloading and installing the Java Development Kit from
Oracle's web site.
Type the code into a text file using a basic text editor like Window's Notepad (Do not use a word processor
because they insert formatting codes into the file), saving the file with the .java file extension. Then
use a batch file to compile the program. The batch file needs to contain the path to the compiler and the
path to the source file, an example of compile.bat is shown below.
c:\Program Files\java\jdk1.8.0_121\bin\javac c:\Users\Stephen\Desktop\program_name.java
pause
The pause command on the second line will cause the DOS shell to stay open until you press any key,
allowing you to read any errors. If successful, the compiler will create an executable file with the
.class file extension. Then use a batch file to run the program. The batch file needs to contain
the start command, the name of the java program launcher, and the name of the compiled java class
file (without the .class extension). An example of run.bat is shown below.
start javaw program_name
The javaw launcher displays the result of your program, or error information if it fails, in a window.
Note: If you wish to retrieve the substring text you can use the String objects substring method, passing
it the index returned from the .indexOf method and the ending index of the substring, which is the starting
index plus the number of characters in the substring.
|