Comparing Two Text Strings in a Java Program
By Stephen Bucaro
One task performed frequently in a Java program is to compare two strings. To accomplish this
you would use the String object's equals method. Shown below is the Java code to compare
the text string "first text" and "second text".
import javax.swing.*;
public class compareText1
{
public static void main(String s[])
{
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.setSize(300,300);
frame.setLocationRelativeTo(null);
frame.setTitle("Compare Text");
String firstPart = "first text";
String secondPart = "second text";
String result = "The strings are the same? " + firstPart.equals(secondPart);
JTextArea myText = new JTextArea();
myText.setLineWrap(true);
myText.append(result);
frame.add(myText);
frame.setVisible(true);
}
}
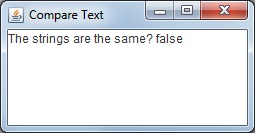
I generally hate it when people add a bunch of extra code to an example. It confuses things.
But in this case I've included the javax.swing library and added code to create a
JFrame and a JTextArea. If I didn't add this extra code, you would need to use
the System.out.print command to output text, and I don't think that's an interesting
or realistic way to learn Java programming. The important part of this code is the line:
String result = "The strings are the same? " + firstPart.equals(secondPart);
Reading from right-to-left this line uses the String object equals method to compare
the two strings and concatenates that with the string "The strings are the same? ". In this
case, since the strings are not the same, it returns false.
Note: unlike in other programming languages, you cannot use the == test, e.g.
if(firstPart == secondPart) in Java to compare strings because that tests for reference
equality (whether they are the same object), not whether they are logically "equal".
If you were to keep the same code, just modifying the text of the second string so that it
is the same as the first string, as shown below, and then compile and run the program again,
it would return the result true.
String firstPart = "first text";
String secondPart = "first text";
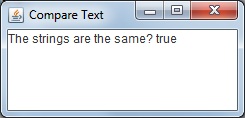
Note: the String object equals method is case-sensitive, if you want to compare
two strings ignoring case, use the equalsIgnoreCase as shown in the code below.
import javax.swing.*;
public class compareText3
{
public static void main(String s[])
{
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.setSize(300,300);
frame.setLocationRelativeTo(null);
frame.setTitle("Compare Text");
String firstPart = "first text";
String secondPart = "First Text";
String result = "The strings are the same? " + firstPart.equalsIgnoreCase(secondPart);
JTextArea myText = new JTextArea();
myText.setLineWrap(true);
myText.append(result);
frame.add(myText);
frame.setVisible(true);
}
}
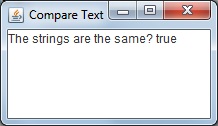
|