Passing Arguments to a Java Application
By Stephen Bucaro
In this article I use a very short example program to demonstrate several basic principles
of Java programming. One is how to pass arguments to a Java application, another is how
to convert a string to an int, and lastly how to use an if structure. The code for this
example is shown below.
public class ageApp
{
public static void main(String[] args)
{
int age = Integer.parseInt(args[0]);
if(age > 18)
{
System.out.println("Age Greater Than 18");
}
}
}
You would save this file as ageApp.java. For this example I compile and run the program using
a DOS batch file under Microsoft Windows. After you have compiled the application into ageApp.class,
the batch file commands to run the application are shown below.
java ageApp 20
pause
You would save this file with the .bat file extension, e.g. run.bat. This batch
file loads the JVM (Java virtual machine) to run the ageApp, passing it the argument 20.
You can add aditional arguments by placing spaces between them. The JVM can accept up to 255
arguments. Note the pause command which keeps the batch file window open until the user
hits a key. Other wise you would never see the results returned by the application.
In the Java code you access the arguments as elements of the args array, the first
argument being args[0]. The args array is an array of strings, so if you need a numerical
value in your application, you need to convert it. The example application passes args[0] to the
Integer.parseInt method to convert it to a primitive int value (as apposed to an Integer object).
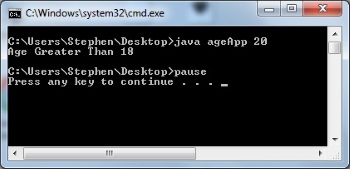
The returned int is placed in the variable age, which is used in an if
structure to test if its value is > (greater than) 18. If the value is greater than 18, the
application displays "Age Greater Than 18".
This is a very simple example, but it deomonstrates how to pass arguments to a Java application,
how to convert a string to an int, and how to use an if structure.
|