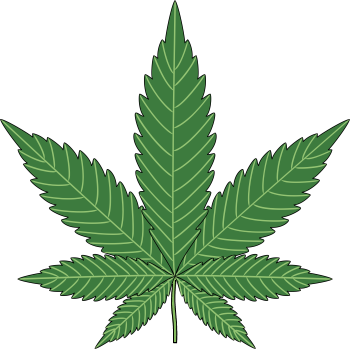
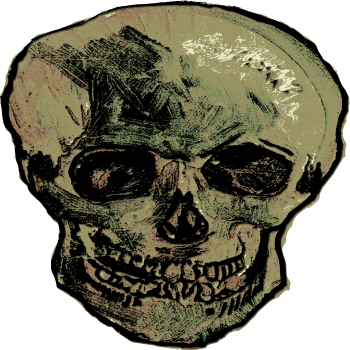
You've probably seen websites where a picture fades out, being replaced by another picture that fades in. This effect is called a visual transition and it's easy to do with just a tiny bit of style and javascript code. But the first thing you need is are the html elements to hold the images in the body of your webpage, this is shown below.
<div style="position:relative; width:350px; height:350px;"> <img id="weed" border="0" width:350px; height:350px; src="marijuana.png" /> <img id="skull" border="0" width:350px; height:350px; src="skull.png" /> </div>
Then comes the style code. The # sign in the selectors shown below selects the elements in the code above with the id "weed" and with the id "skull". Setting their postion properties to absolute, along with setting their left and top properties to 0px places the images directly on top of one another.
<style> #weed { position: absolute; left: 0px; top: 0px; opacity: 1; transition: opacity 3s; } #skull { position: absolute; left: 0px; top: 0px; opacity: 0; transition: opacity 3s; } </style>
Note that the opacity property of one image is set to 1 (totally opaque) and the opacity property of the other image is set to 0 (totally transparent). The transition property is set to the property which the transition will apply to (opacity), and to the duration of the transition (3 seconds). Paste this style code block into the head section of your webpage.
Lastly, we need some JavaScript code to switch the opacity values of the two images. The code for this is shown below. First we declare a flag named vis, then we set an interval timer that calls the function myTrans every 6 seconds. (6000 milliseconds).
<script> var vis = 0; var myVar = setInterval(myTrans, 6000); function myTrans() { if(vis == 0) { fadeWeed(); vis = 1; } else { fadeSkull() vis = 0; } } function fadeWeed() { weed.style.opacity = 0; skull.style.opacity = 1; } function fadeSkull() { weed.style.opacity = 1; skull.style.opacity = 0; } </script>
The function myTrans has an if statement that checks the flag. If the flag is 0, it calls the fadeWeed() function and sets the flag to 1. If the flag is 1, it calls the fadeSkull() function and sets the flag to 0.
The fadeWeed() function sets the opacity of the image with the id weed to 0, and the opacity of the image with the id skull to 1. The fadeSkull() function does just the opposite, setting the opacity of the image with the id skull to 0, and the opacity of the image with the id weed to 1. The time interval for this change to take place is set to 3 seconds in the styles, so it wouldn't make sense for the interval timer to be shorter than that.
As you can see, the code to fade between two different pictures is very simple. You can easily modify the code to work with your images and your transition and interval times. This code should also be easy to modify to fade between more than two images.
More Java Script Code:
• Easy Rollovers
• Learn JavaScript Visually
• Easy HTML5 Drag and Drop
• JavaScript Code to Add or Remove Specific Elements of an Array
• Code for Java Script Circle/Sphere Calculator
• Replace Drop-down with Drag-and-drop
• Create Your Own Database Using Only Notepad : CDV
• Using the Java Script Array Object
• Web Site Menus : Which Section Am I In?
• Easy Slide Show Code with Mouseover Pause